Structure from Motion
This project aims to estimate structure (3D position for sparse scene points) and motion (camera parameters for images), given a sequence of images.
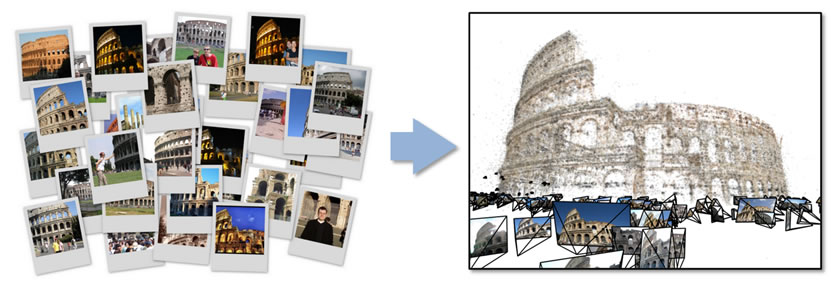
Getting Started
Preliminaries
Planar Transformation
Camera Calibration
Two-view Reconstruction
Multi-view Reconstruction